Making HTTP calls to your mock API server using React
Learn how you can call your REST API from your React application and how to mock it using Mockfly as a mock API server.
This guide will teach you how to call any API from React using fetch.
We're going to simulate a tasks API, where we'll fetch the list of tasks and render them on the screen using React.
Creating the mock endpoint with Mockfly
First, we need to create a mock endpoint. In this case, it will be a mock endpoint using the GET verb that will return a list of tasks. This is very useful for simulating a real API without having to wait for it to be available. You can read more about what and why it's a good idea to use a mock server in this article.
To do this, we'll create a mock endpoint in Mockfly with the route /tasks and set it as a GET type. For the body, we'll use Mockfly's AI to generate a list of random tasks to mock the response body. Simply write in the body what we want the AI to return. If you want to learn more about using AI in your mock server, you can read this article.
Therefore, by simply writing: //a list of tasks in the body, we would automatically have a body with a list of tasks like this:
{
"tasks": [
{
"id": 1,
"title": "Buy groceries",
"completed": false
},
{
"id": 2,
"title": "Finish report",
"completed": true
},
{
"id": 3,
"title": "Go to the gym",
"completed": false
}
]
}
Calling our mock endpoint from React
Now that we have our mock endpoint created to return a list of tasks, we need to make the call to this mock endpoint from our React application. For this, we need to know the base URL of our mock server and the route of our endpoint, which in this case is /tasks. We can directly copy it from the input provided by Mockfly:
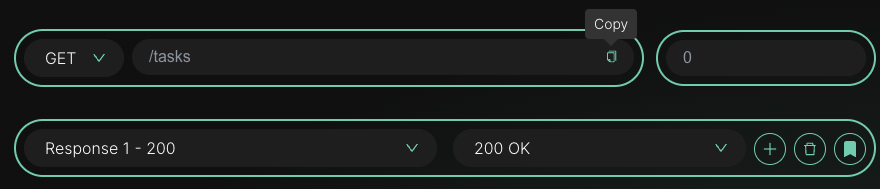
Once we have this, we can create a React component that makes the call to our mock endpoint and renders the tasks it returns on the screen. To do this, we'll do something like this:
const Tasks = () => {
const [tasks, setTasks] = useState([])
useEffect(() => {
fetch('https://api.mockfly.dev/mocks/{{your-project-slug}}/tasks')
.then(response => response.json())
.then(json => {
setTasks(json.tasks)
})
}, [])
return (
<div>
<h1>Tasks</h1>
<ul>
{tasks?.map(task => (
<li key={task.id}>{task.title}</li>
))}
</ul>
</div>
)
}
In this code, we're calling our mock endpoint using fetch within the useEffect and rendering the tasks on the screen. The mock API returns a JSON where we have an array of tasks, so we just need to update the state using setTasks with the array of tasks returned by the mock API and then iterate over the tasks array to render the title.